- RESOURCES -
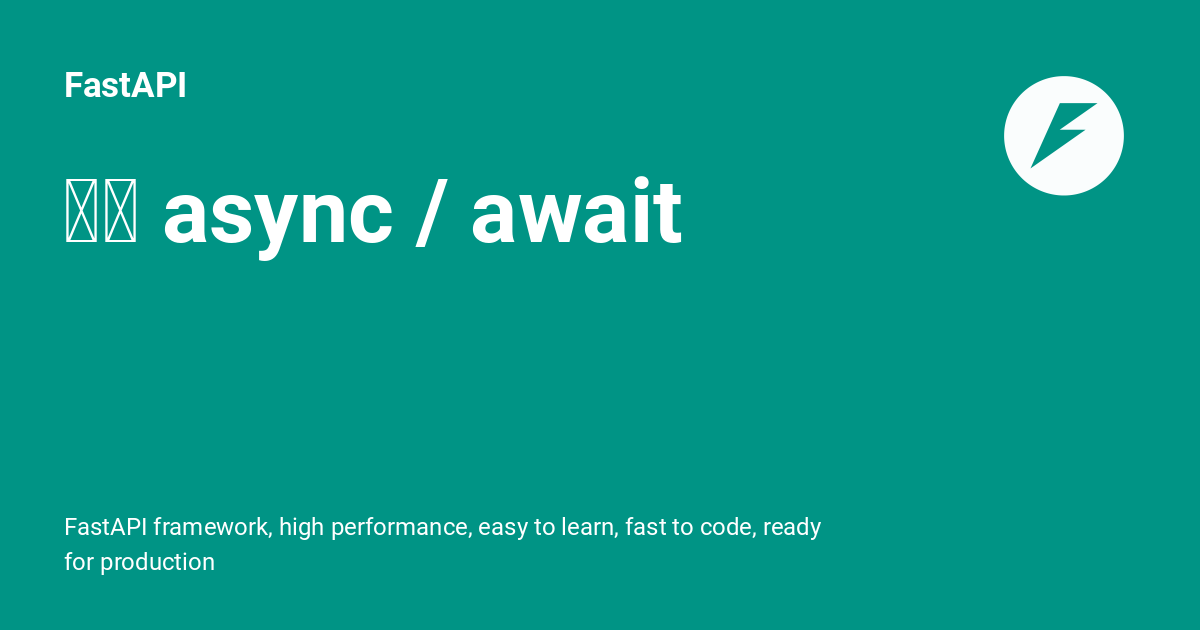
并发 async / await - FastAPI
FastAPI framework, high performance, easy to learn, fast to code, ready for production......
fastapi.tiangolo.com

FastAPI 多线程使用教程,提升代码运行性能_牛客网
在现代网络应用中,高性能和快速响应是至关重要的,Python 的 FastAPI 框架以其出色的性能和简单易用的特点,成为了许多开发者的首选。然而,在某些场景下,单线程运行可能无法满足需求,这时候就需要考虑使用多线程来提高应用的并发性能。本文将介绍 FastAPI 框架中多线程的使用方法,包括常见需_牛客网_牛客在手,offer不愁......
www.nowcoder.com
fastapi 如何控制并发——其一_fastapi高并发-CSDN博客
文章浏览阅读961次,点赞17次,收藏18次。先说结论,单独靠这里的业务场景是:单机只启动一个进程,也就是,同时只能只处理一个请求,其他的请求全部拒绝,而不进行排队。我们使用了fastapi。_fastapi高并发......
blog.csdn.net
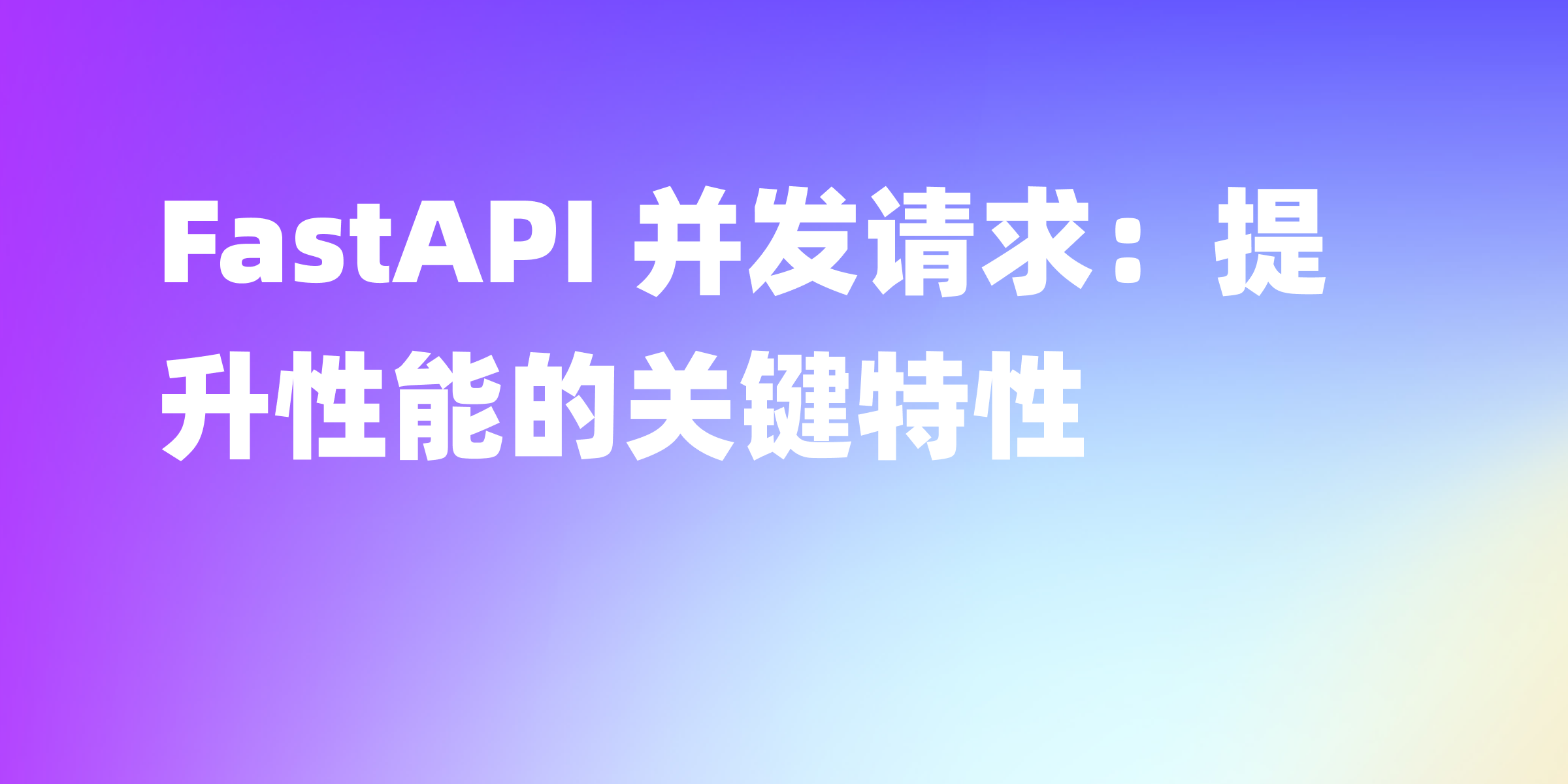
FastAPI 并发请求:提升性能的关键特性
本文介绍了如何使用 FastAPI 实现并发请求......
apifox.com

并发和异步/等待 - FastAPI 中文
......
fastapi.org.cn
FastAPI 并发请求详解:提升性能的关键特性-CSDN博客
文章浏览阅读1.8k次,点赞20次,收藏22次。本文详细介绍了FastAPI在处理高速响应和并行请求方面的优势,通过示例展示了如何使用异步编程和asyncio库,以及如何使用uvicorn启动和调试FastAPI应用。......
blog.csdn.net
fastapi 如何控制并发——其三_fastapi 并发数-CSDN博客
文章浏览阅读286次,点赞4次,收藏10次。随后我们改用多进程的方式去测试,在客户端并发数为4,服务端进程数为8的情况下,平均耗时也有347ms,并且成功率只有22%,这里的347s存在水分,很多失败的请求只有1~2ms,存在摊还的情况。那可能真的是在并发数上来的情况下确实是耗时增大了,我们猜测是并发多了后,CPU成为了瓶颈,我们在使用多进程方案时,观察CPU利用率,发现确实已经占满了。上面我们使用的是多线程编程,正如我们之前所说的,对于计算密集型服务,python的多进程就是个笑话,这是由于。......
blog.csdn.net

体验 Python FastAPI 的并发能力及线, 进程模型 | 隔叶黄莺 Yanbin Blog - 软件编程实践
本文进行实际测试 FastAPI 的并发能力,即同时能处理多少个请求,另外还能接收多少请求放在等待队列当中; 并找到如何改变默认并发数; 以及它是如何运用线程或进程来处理请求。我们可以此与 Flask 进行对比,参考 Python Flask 框架的并发能力及线,进程模型,是否真如传说中所说的 FastAPI 性能比 Flask 强, FastAPI 是否对得起它那道闪电的 Logo。 本文使用 JMeter 进行测试,测试机器为 MacBook Pro, CPU 6 核超线程,内存 16 Gb......
yanbin.blog
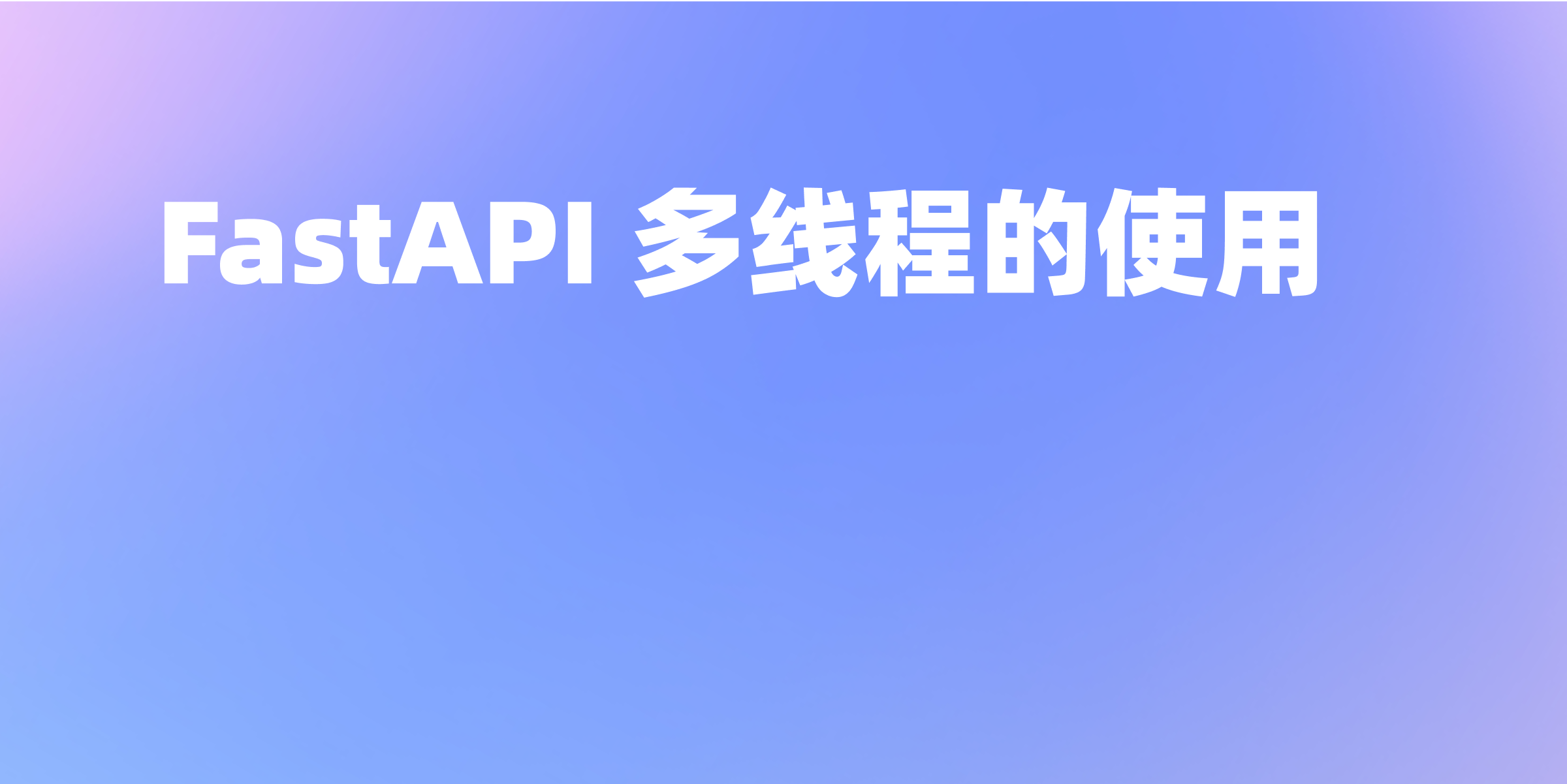
FastAPI 多线程的使用:提升代码运行性能
本文介绍了FastAPI框架中多线程的使用方法。......
apifox.com