- RESOURCES -
Selenium打开网页时保持登陆状态_selenium保持登录状态-CSDN博客
文章浏览阅读8.3k次,点赞6次,收藏21次。本文介绍了如何使用Selenium在打开网页时直接处于登录状态,避免每次手动登录。通过设置ChromeOptions,指定用户数据目录,可以加载已登录的Chrome配置,从而实现自动化测试时的登录状态保持。这种方法适用于需要频繁测试已登录功能的场景。......
blog.csdn.net
python - 在 Python 中使用 Selenium 保持登录状态-6ren
python - 在 Python 中使用 Selenium 保持登录状态-我正在尝试登录一个网站,然后在登录后使用 Selenium 导航到保持登录状态的网站上的另一个页面。然而,当我尝试导航到不同的页面时,我发现我已经注销了。 我相信这是因为我不明白 webdriver.......
123.56.139.157
request库如何用pickle保存和读取cookie供给下次直接模拟登录_requests session pickle-CSDN博客
文章浏览阅读648次。作者:虚坏叔叔博客:https://xuhss.com早餐店不会开到晚上,想吃的人早就来了!😄一、保存cookie文件import picklesession = requests.session()res = session.post(url,data=post_data, headers = headers)coolie_jar = res.cookies.get_dict()with open("douban.cookie", "wb") as f: pickle.d......
blog.csdn.net
使用selenium打开网页并保存cookie至json文件_怎么导出谷歌浏览器的cookie为json-CSDN博客
文章浏览阅读2.1k次。在编写爬虫,或者模拟浏览器进行一些操作时,如果每次都模拟用户输入账号密码登陆,实现步骤较为复杂,登陆后抓取cookies,在后续操作中使用cookies登陆是一种较为简便的方法。因此,本文使用python3 + chromedriver,帮助完成cookies的抓取。需要安装python3环境,安装selenium和json包,并下载对应版本的chromedriverfrom selenium import webdriverimport json options = w......
blog.csdn.net
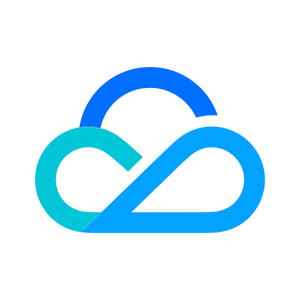
Selenium:添加Cookie的方法-腾讯云开发者社区-腾讯云
从中可以看出add_cookie()这个函数有一个参数cookie_dict,它是以字典的形式传入的,字典中必选的键是"name"和"value",可选的键是"path", "domin", "secure", "expiry",其实源码中还漏了一个:"httponly"。......
cloud.tencent.com
selenium设置chrome浏览器保持登录方式两种options和cookie - 后来的后来 - 博客园
方法一: 1.在初始化driver之前先设置options 2.其他关于options介绍链接:http://www.cnblogs.com/baoyu7yi/p/7058537.html 3.代码 方法二: 1.通过F12查找到保存登录的cookie 2.cookie添加的源码解读 由源码可以看出......
www.cnblogs.com
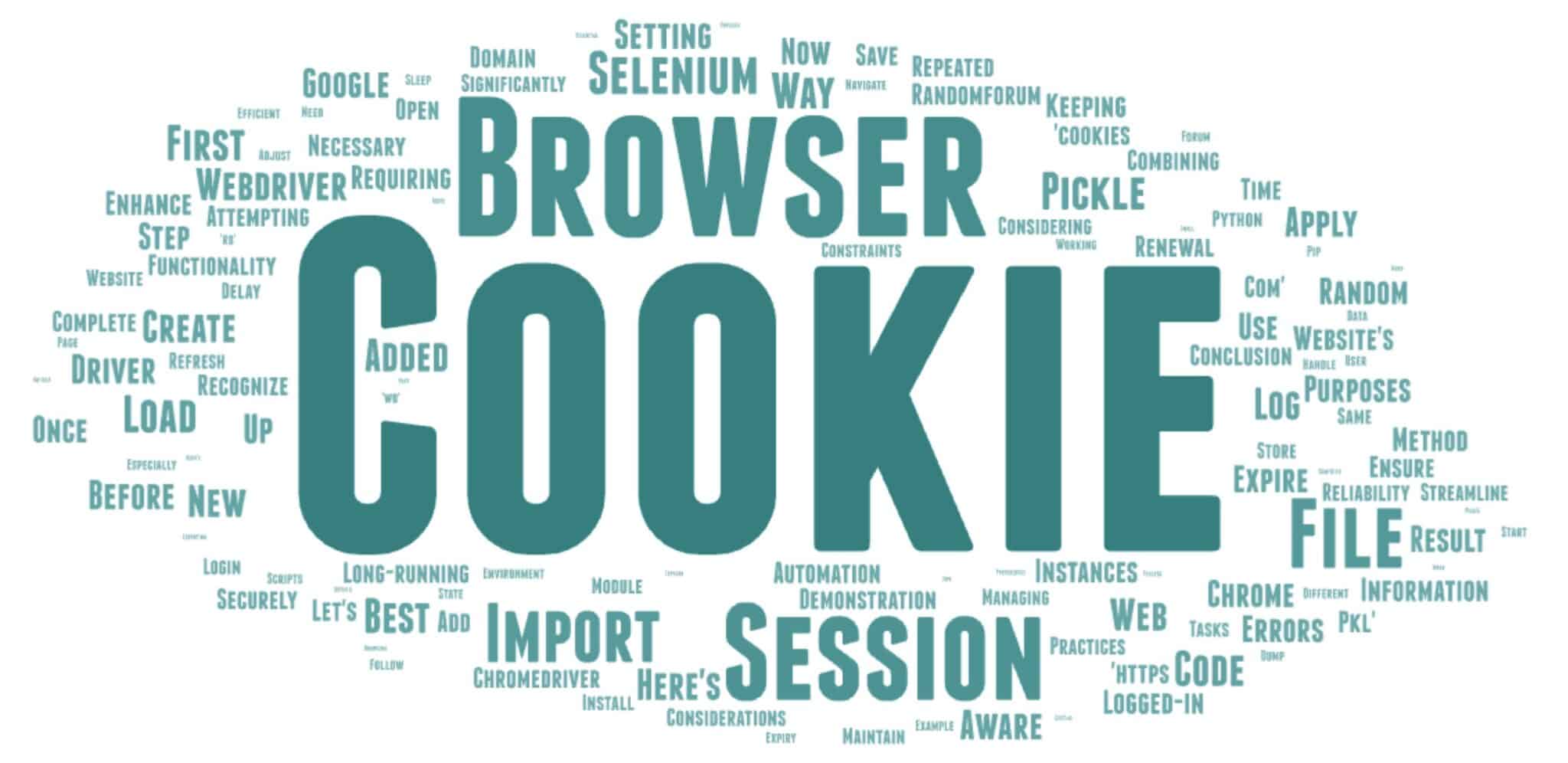
使用 Selenium 和 Python 进行有效的 Cookie 管理:导入和导出 Cookie
了解如何使用 Selenium 和 Python 在 Web 自动化中高效处理 Cookie。掌握导入和导出 Cookie 的技巧,以增强控制。......
fineproxy.org
Pickle 保存和读取Cookie实现免登陆_cookies = pickle.load(open('cookies.pkl', 'rb'))-CSDN博客
文章浏览阅读1.7k次。Pickle 保存和读取Cookie实现免登陆from selenium import webdriverfrom time import sleepimport pickleimport tracebackdriver = webdriver.Firefox(r"E:\43j6ugol.d")driver.get("https://wj.qq.com/")sleep(40_cookies = pickle.load(open('cookies.pkl', 'rb'))......
blog.csdn.net
保存和加载模型 — PyTorch 教程 2.4.0+cu124 文档 - PyTorch 中文
......
pytorch.ac.cn
python selenium如何保存网站的cookie用于下次自动登录_senlium 登录保存cookie-CSDN博客
文章浏览阅读7.4k次,点赞6次,收藏11次。# 一、python selenium如何保存网站的cookie使用Selenium保存网站的Cookie非常简单。在这个示例中,我们使用Chrome浏览器和Chrome WebDriver,你可以根据需要更改为其他浏览器和相应的WebDriver。这段代码的关键部分在于使用来获取当前页面的所有Cookie,并将其保存到一个文本文件中。每个Cookie被写入文件的一行,格式为。你可以将文件名和路径更改为你喜欢的位置。请注意,保存的Cookie在未来可......
blog.csdn.net